
Insert → To insert a new element in the queue.Ģ. There are mainly 4 operations we want from a priority queue:ġ. We use a max-heap for a max-priority queue and a min-heap for a min-priority queue. Heaps are great for implementing a priority queue because of the largest and smallest element at the root of the tree for a max-heap and a min-heap respectively. It is also used in scheduling processes for a computer, etc. Priority queues are used in many algorithms like Huffman Codes, Prim's algorithm, etc.
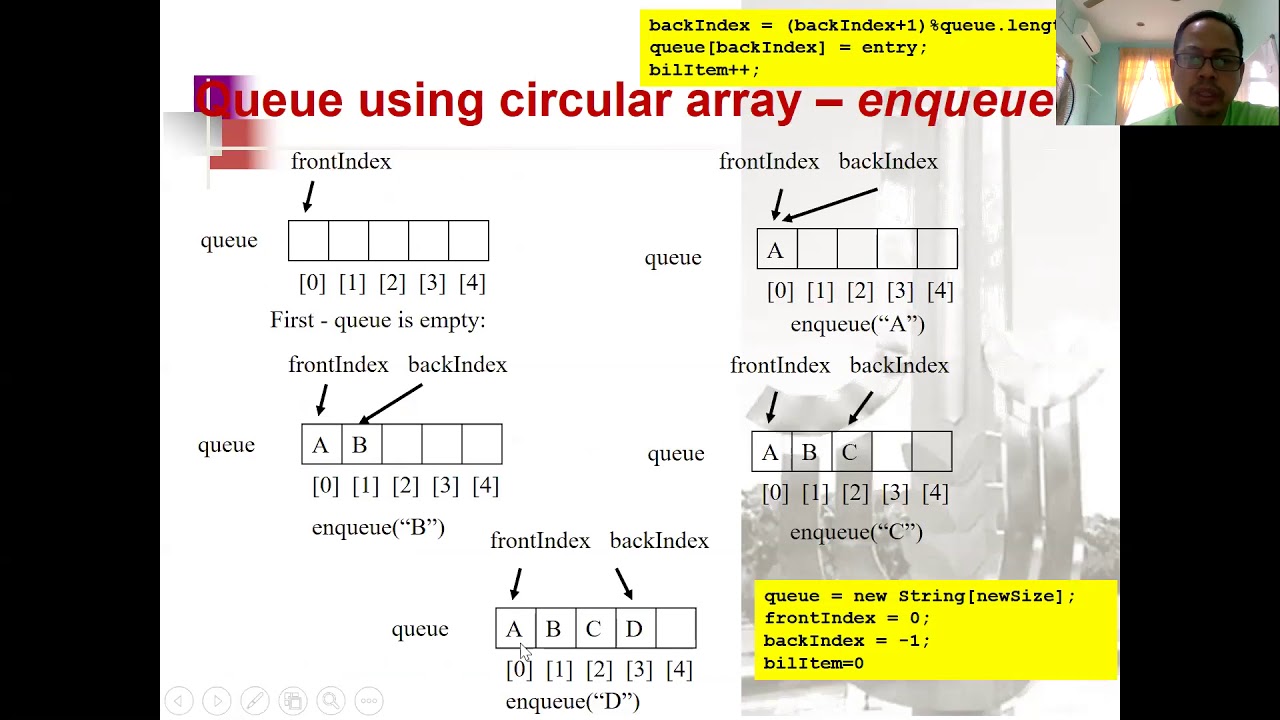
Thus, a max-priority queue returns the element with maximum key first whereas, a min-priority queue returns the element with the smallest key first. ∗/ public interface PriorityQueue /** Returns the Position of an entry having minimal key.Priority queue is a type of queue in which every element has a key associated to it and the queue returns the element according to these keys, unlike the traditional queue which works on first come first serve basis. ∗∗ Interface for the priority queue ADT. Transitive property: if k1 ≤ k2 and k2 ≤ k3, then k1 ≤ k3.Antisymmetric property: if k1 ≤ k2 and k2 ≤ k1, then k1 = k2.Comparability property: k1 ≤ k2 or k2 ≤ k1.it satisfies the following properties for any keys k1, k2, and k3:.Returns a boolean indicating whether the priority queue is empty.Returns the number of entries in the priority queue.Removes and returns an entry (k,v) having minimal key fromm the priority queue.returns null if the priority queue is empty.Returns (but does not remove) a priority queue entry (k,v) having minimal key.Creates an entry with key k and value v in the priority queue.different financial analysts may assign different ratings (i.e., priorities) to a particular asset,.The element with the minimal key will be the next to be removed from the queue (thus, an element with key 1 will be given priority over an element with key 2).When an element is added to a priority queue, the user designates its priority by providing an associated key.A binary heap will allow us both enqueue and dequeue items in 𝑂(log𝑛).The classic way to implement a priority queue is using a data structure called a binary heap.

However, inserting into a list is 𝑂(𝑛) and sorting a list is 𝑂(𝑛log𝑛).Implement a priority queue using sorting functions and lists. different financial analysts may assign different ratings (i.e., priorities) to a particular asset, such as a share of stock.With such generality, applications may develop their own notion of priority for each element.Although it is quite common for priorities to be expressed numerically, any Java object may be used as a key, as long as there exists means to compare any two instances a and b, in a way that defines a natural order of the keys.an element with key 1 will be given priority over an element with key 2.The element with the minimal key will be the next to be removed from the queue.the user designates its priority by providing an associated key.When an element is added to a priority queue Thus when you enqueue an item on a priority queue, the new item may move all the way to the front.The highest priority items are at the front of the queue and the lowest priority items are at the back.However, in a priority queue the logical order of items inside a queue is determined by their priority.A priority queue acts like a queue that dequeue an item by removing it from the front.and allows the removal of the element that has first priority.a collection of prioritized elements that.“first come, first serve” policy might seem reasonable, yet for which other priorities come into play.It is unlikely that the landing decisions are based purely on a FIFO policy.In practice, there are many applications in which a queue-like structure is used to manage objects that must be processed in some way, but the first-in, first-out policy does not suffice. Data Structures and Algorithms in Java, 6th Edition.pdf.Implementing an Adaptable Priority Queue.Asymptotic Analysis of Bottom-Up Heap Construction.

Implementing a Priority Queue with a Heap.UnsortedPriorityQueue class Unsorted List.AbstractPriorityQueue Abstract base class.Data Structures Basic 1 Priority Queues.
